Alright, so I kept hearing about this Julia language. People saying it’s fast, easy like Python, good for science stuff. Sounded interesting enough, so I thought, why not give it a try? Needed a small project, something visual maybe, just to kick the tires.
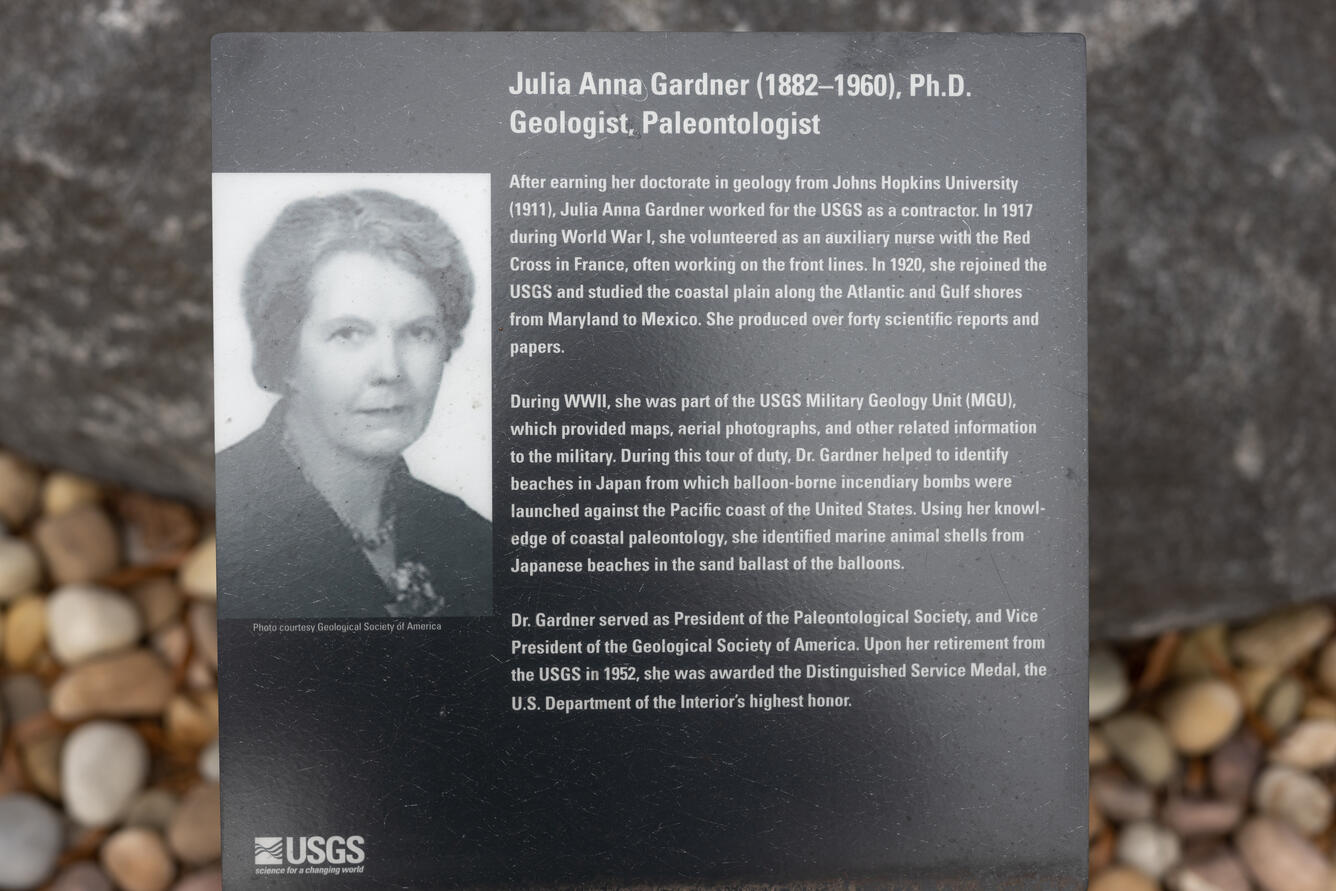
Figuring Out What To Do
I settled on making one of those simple falling sand things. You know, pixels that drop down and pile up. Seemed like a decent test. Involved arrays, loops, maybe some simple graphics. Shouldn’t be too hard, right? That’s what I thought, anyway.
Getting Started – The Setup
First step, obviously, was getting Julia installed. Went to their site, downloaded the installer for my system. That part was pretty smooth, gotta say. Ran the installer, and boom, Julia was there. Opened up the terminal, typed julia
, got the prompt. Okay, phase one complete.
Next, I needed a way to actually see the sand. Just printing numbers wasn’t gonna cut it. I poked around online, looked for simple plotting or graphics libraries. Found something called . Seemed popular enough. Getting that installed took a bit more time. Had to run the package manager commands inside Julia:
using Pkg
*("Plots")
It downloaded a bunch of stuff. Took a few minutes. Then I tried using Plots
and it spent ages precompiling. Felt like watching paint dry. Seriously long wait the first time. But okay, eventually it finished. Now I supposedly had the tools.
Writing The Actual Code
Now for the “sand” part. I decided to use a 2D array, maybe fill it with zeros (empty) and ones (sand). The logic seemed straightforward:
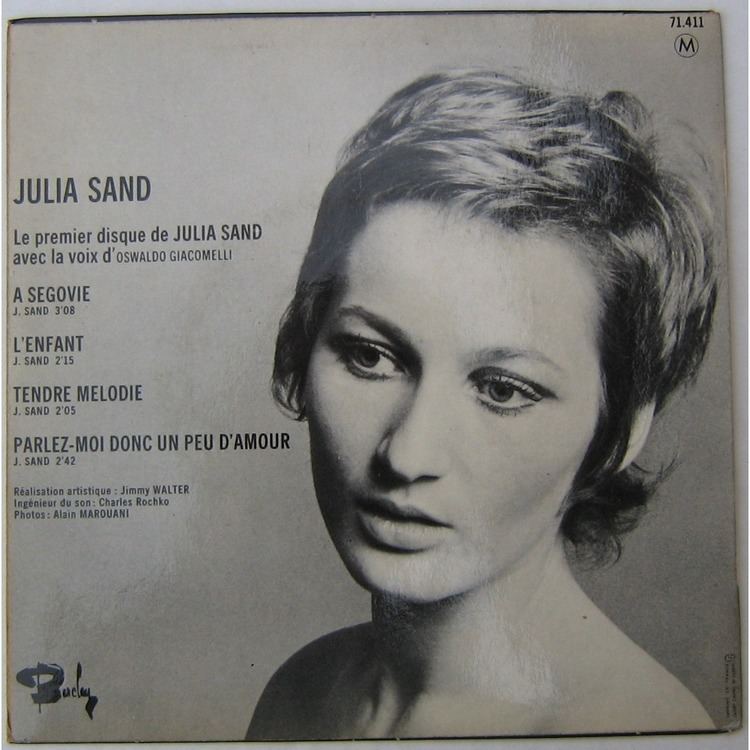
- Loop through each spot in the grid from bottom to top.
- If you find sand (a ‘1’), check the spot directly below it.
- If the spot below is empty (a ‘0’), move the sand down. Swap the ‘1’ and ‘0’.
- Maybe add a check for falling slightly left or right if directly below is blocked.
- Add new sand particles at the top occasionally.
Started coding this up. The Julia syntax felt a bit weird at first, kinda like a mix of Python and MATLAB maybe? Indexing starts at 1, not 0. That tripped me up a couple of times. Loops looked familiar enough, for
loops and if
statements.
Got the basic gravity working. Sand fell straight down. But then updating the array in place while looping caused problems. Sand particles were moving multiple steps in one frame. Had to make a copy of the grid or be more careful with the loop order. Went with iterating bottom-up, right-to-left, that seemed to fix the multi-step issue.
Then tried adding the diagonal fall. If below is blocked, check bottom-left, then bottom-right. Pick one randomly if both are free. This made the piling look a bit more natural.
Making it Visual (Sort of)
Getting * to just show the grid as an image wasn’t immediately obvious to me. I fiddled around with heatmap functions. heatmap(grid)
kinda worked. It showed the array, different colors for 0 and 1. Needed to update it in a loop to see the animation.
Putting it in a loop and calling display(heatmap(...))
repeatedly was super slow at first. The whole plot window flickered, redrew completely each time. Not exactly the smooth animation I pictured. Maybe * wasn’t the best tool for real-time simple pixel stuff? Probably needed something lower level, like maybe * or a simple SDL wrapper, but I wanted to stick with what I’d already installed for this first try.
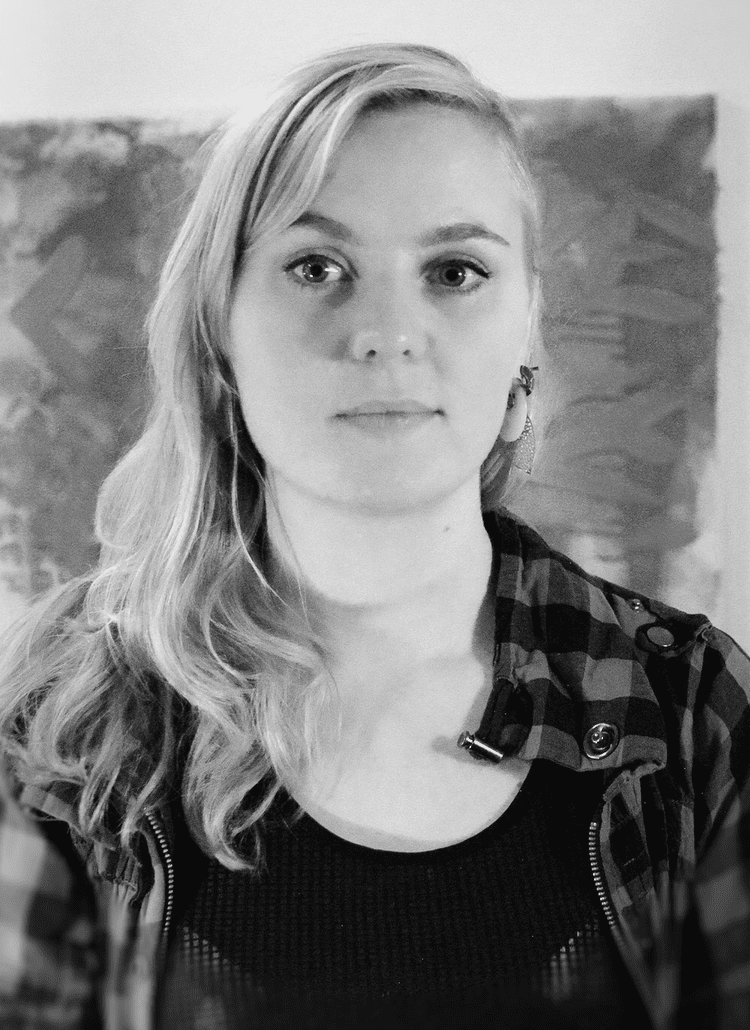
I tweaked the plot command, trying different options. Found some way to update the plot data more efficiently, but it still wasn’t great. Good enough to see the sand piling up, though.
Final Thoughts
So, did I get a fancy sand simulation running? Not really. I got pixels falling down and forming piles in a slow-updating plot window. It worked, technically.
What did I learn? Julia itself seems okay. The syntax is manageable once you get used to the 1-based indexing and the end
keyword everywhere. The package manager is built-in, which is nice. That initial precompile time for * was annoying, though. And for simple, fast, real-time graphics, * felt like the wrong tool for the job – too heavy.
Was it worth the effort? Yeah, I guess. Got my feet wet with Julia. Saw some potential, especially the talk about speed (though my little script wasn’t exactly pushing performance limits). But for just whipping up a quick visual demo like this, maybe sticking to Python with Pygame or Processing would have been faster to get something satisfying on screen. Still, interesting experiment.