Alright, let me tell you about my little Hanoi, or Ho Chi Minh, adventure. It all started last week when I was fiddling around with some recursion stuff. You know, just trying to keep the brain cells firing.
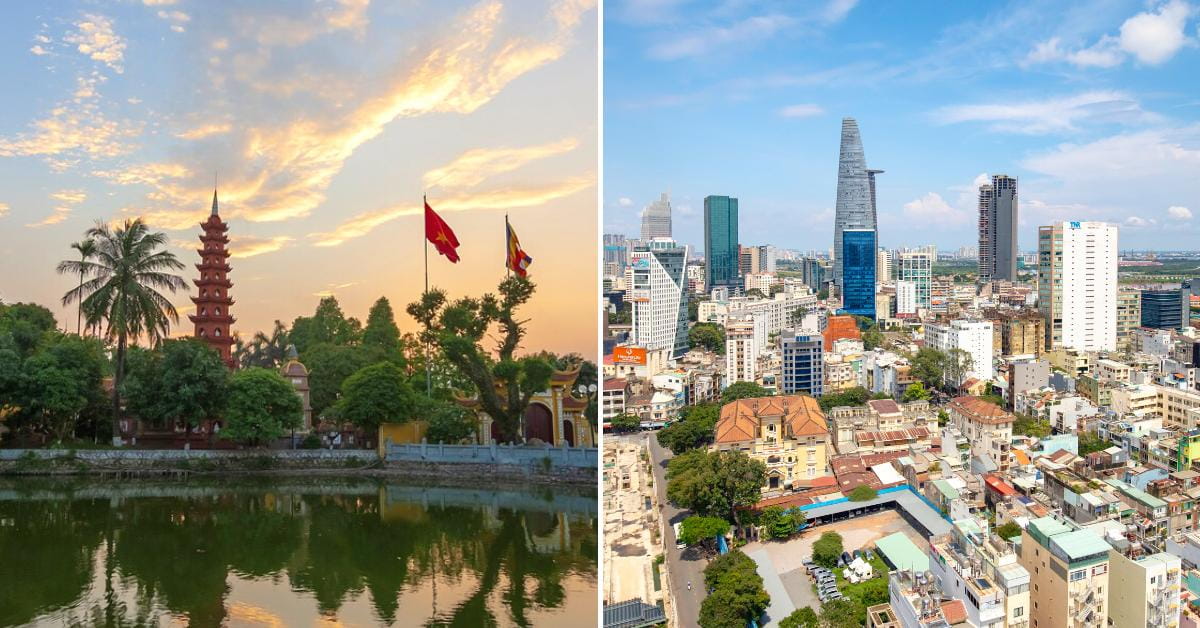
So, the Hanoi Tower problem pops into my head. Classic CS stuff, right? I hadn’t messed with it since, like, college. I figured, “Hey, why not give it a whirl in Python?” I fired up my trusty VS Code and just started banging out code.
First things first, I defined the function: def hanoi(n, source, destination, auxiliary):. That’s where the magic should happen. ‘n’ for the number of disks, and then the source, destination, and auxiliary pegs. Pretty standard.
Now, for the recursion. This is where it gets kinda brain-twisty. The base case is easy: if n == 1:. If there’s only one disk, just move it. I printed something like print(f”Move disk 1 from {source} to {destination}”). Simple enough.
Then came the recursive steps. I swear, every time I look at this problem, I gotta re-think it. But eventually I got it down:
- Move the top n-1 disks from source to auxiliary peg: hanoi(n-1, source, auxiliary, destination)
- Move the nth disk from source to destination peg: print(f”Move disk {n} from {source} to {destination}”)
- Move the n-1 disks from auxiliary peg to destination peg: hanoi(n-1, auxiliary, destination, source)
I plugged that all into the function. I had to double-check the order of the pegs in the recursive calls a bunch of times. Getting those mixed up is a recipe for disaster.
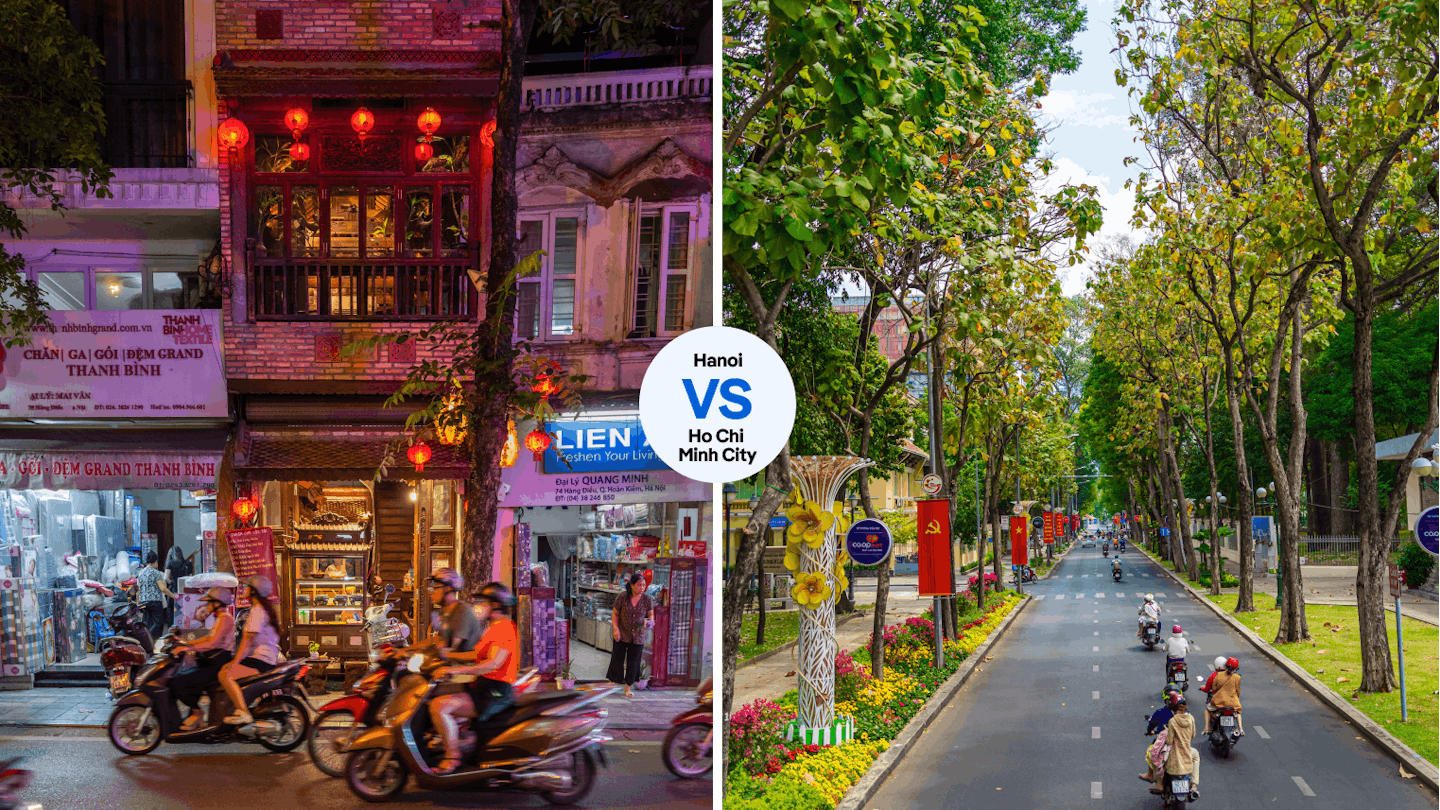
To test it, I just did something simple: hanoi(3, ‘A’, ‘C’, ‘B’). That’s 3 disks, moving from peg A to peg C, using peg B as the helper. I ran it, and BAM! It spat out all the steps, looking just like it should.
But here’s the thing, just making it work wasn’t enough. I wanted to visualize it. I thought, “Okay, I’m gonna use a library.” But which one? Matplotlib? Nah, too much overhead for something so simple. I didn’t wanna overcomplicate things.
So, I decided to stick with the bare minimum. I figured I could represent the disks as numbers, and the pegs as lists. Then, I could just print the state of the pegs after each move.
I created a new function, def hanoi_visual(n, source, destination, auxiliary):. This time, instead of just printing move instructions, it would actually manipulate the disk lists and print the pegs.
I initialized the pegs: source = list(range(n, 0, -1)), destination = [], and auxiliary = []. This puts the disks in order on the source peg. Now, inside the recursive function, instead of printing, I did actual moves: disk = *(), *(disk).
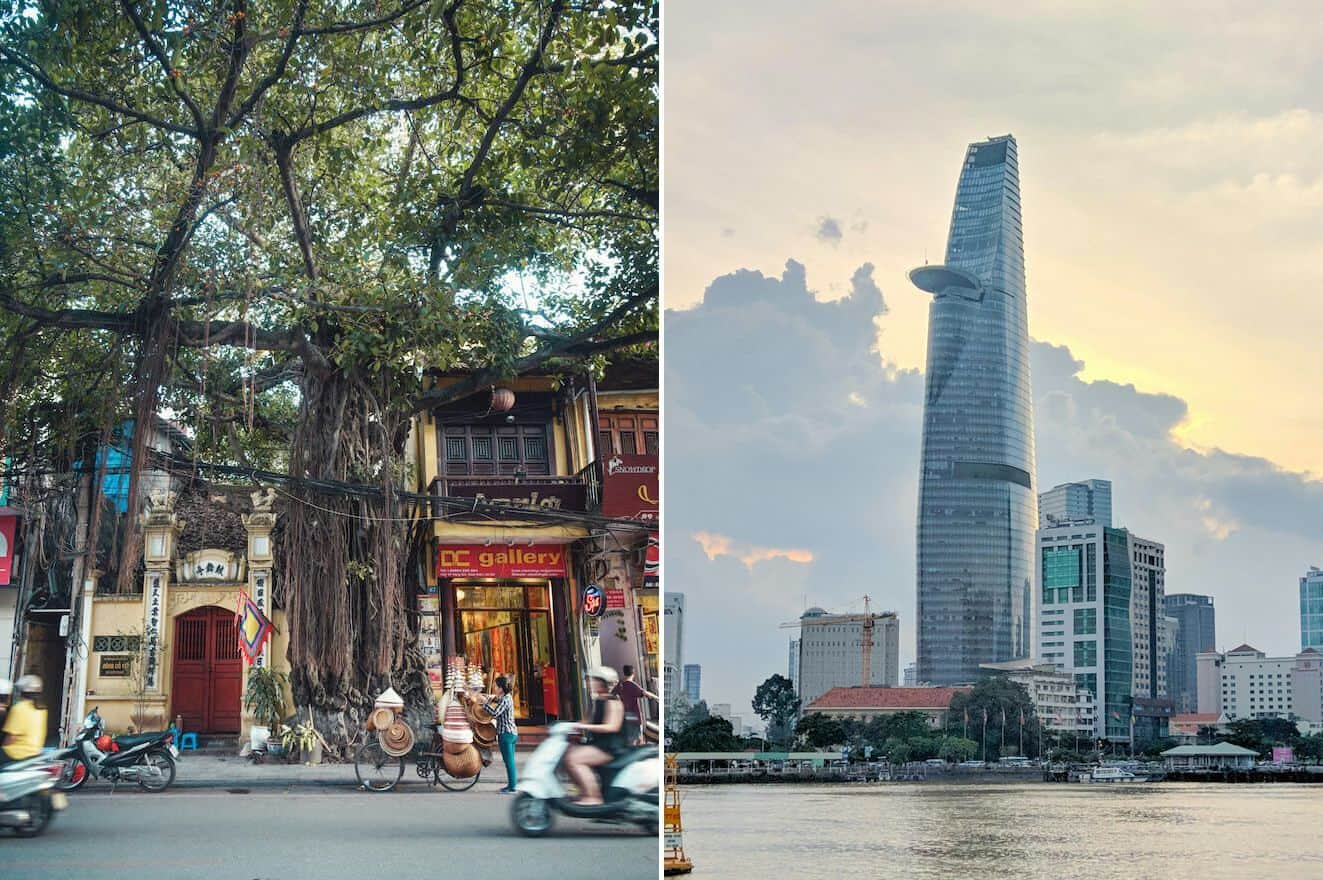
After each move, I printed the states of the pegs. Something like: print(f”Source: {source}, Destination: {destination}, Auxiliary: {auxiliary}”).
It was still a text-based visualization, but way more informative than just the move instructions. I could see the disks moving from peg to peg.
Then, to make it even more interesting, I added a little time delay using *(1) after each move. This slowed things down enough that I could actually follow the moves in real-time. It was kinda mesmerizing, watching the disks shuffle back and forth.
Now, why did I call it “Hanoi or Ho Chi Minh”? Honestly, just because it popped into my head and sounded vaguely interesting. No deep meaning there. Just a random thought while debugging.
In the end, it was a fun little project. I re-familiarized myself with recursion, and got a slightly better handle on visualizing algorithms. Not bad for a few hours of coding on a lazy afternoon!
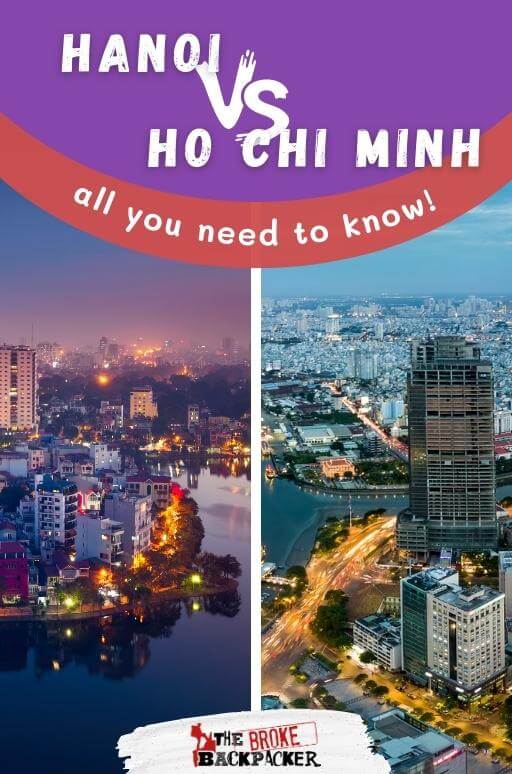