Okay, so today I’m gonna walk you through this little “works flash” thing I’ve been messing around with. It’s not perfect, but it gets the job done, and I learned a bunch along the way.
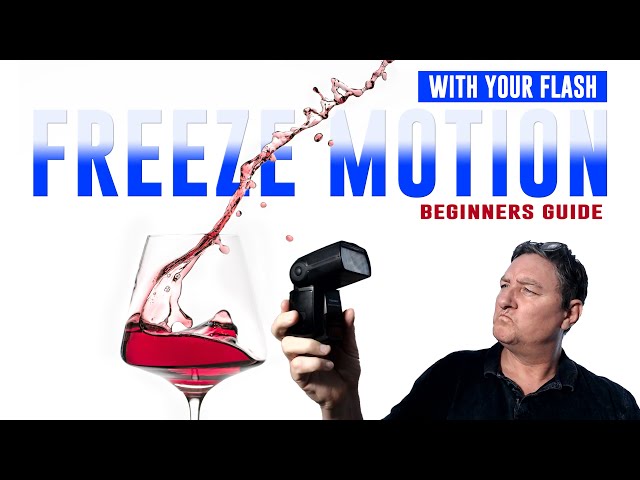
The Idea
Basically, I wanted a quick way to, like, flash certain actions or processes are ongoing. Think progress bars, but way simpler and more visual. I needed something that could grab attention, especially when some backend stuff was chugging away.
Getting Started: The Initial Brainstorm
First, I just scribbled some ideas on a notepad. I figured I’d use JavaScript, since I’m somewhat comfortable with it, and CSS for styling. I started with a basic HTML structure:
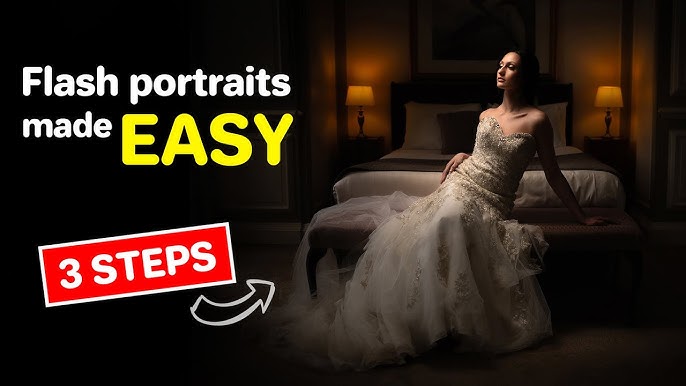
Simple, right? The `flash-container` is just a wrapper, and `flash-bar` is what’s gonna do the flashing.
CSS Styling: Making it Flash!
Next up was the CSS. I wanted a bright, noticeable color. Here’s what I came up with:
css
#flash-container {
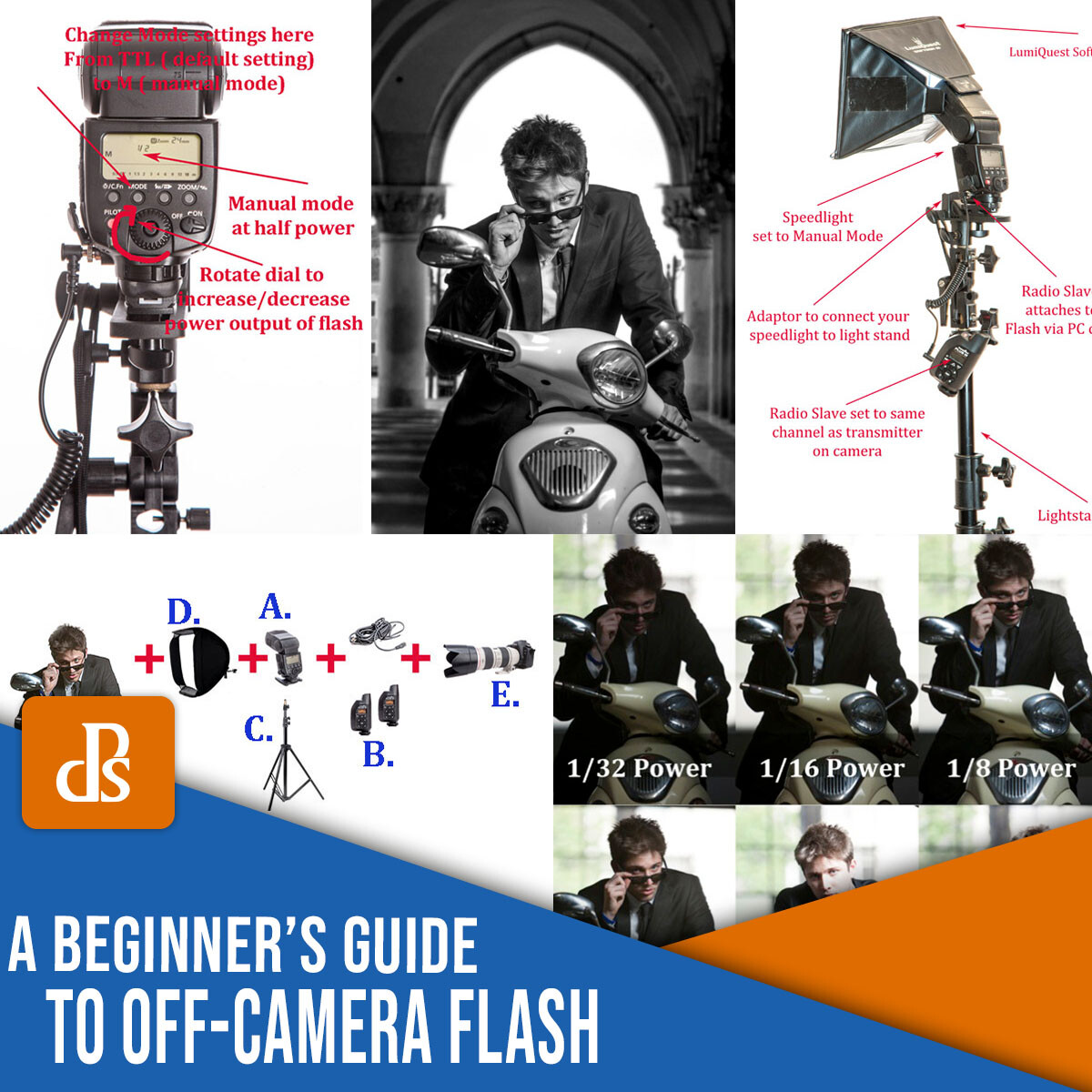
width: 100%;
height: 10px; / Adjust as needed /
background-color: #eee; / A light background /
overflow: hidden; / Important! /
#flash-bar {
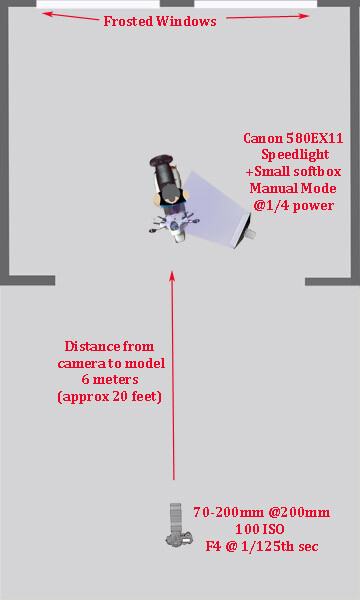
width: 0%; / Starts empty /
height: 100%;
background-color: #ff4d4d; / Bright red! /
animation: flash 1.5s linear infinite;
@keyframes flash {
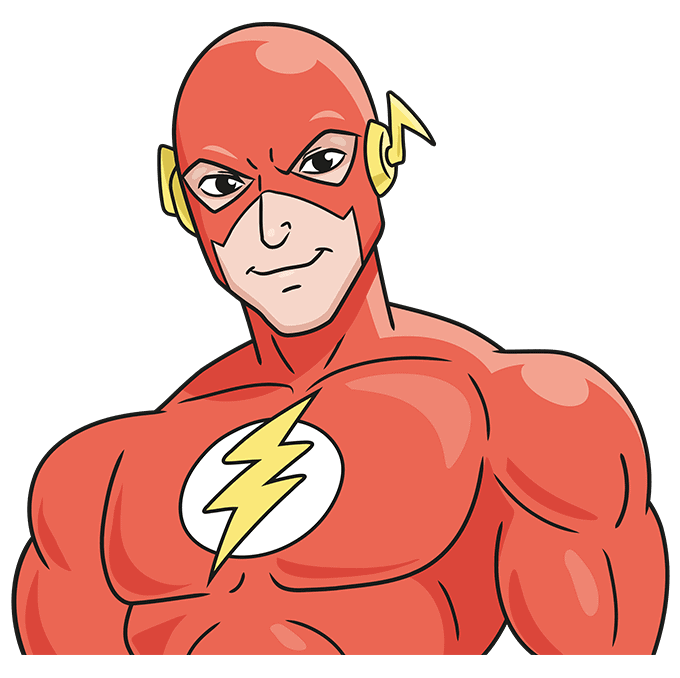
0% { width: 0%; }
50% { width: 100%; }
100% { width: 0%; }
The key here is the `@keyframes flash` animation. It makes the `flash-bar` expand to 100% width and then back to 0%, creating the flashing effect. The `overflow: hidden` on the container is crucial; otherwise, the overflowing `flash-bar` would be visible.
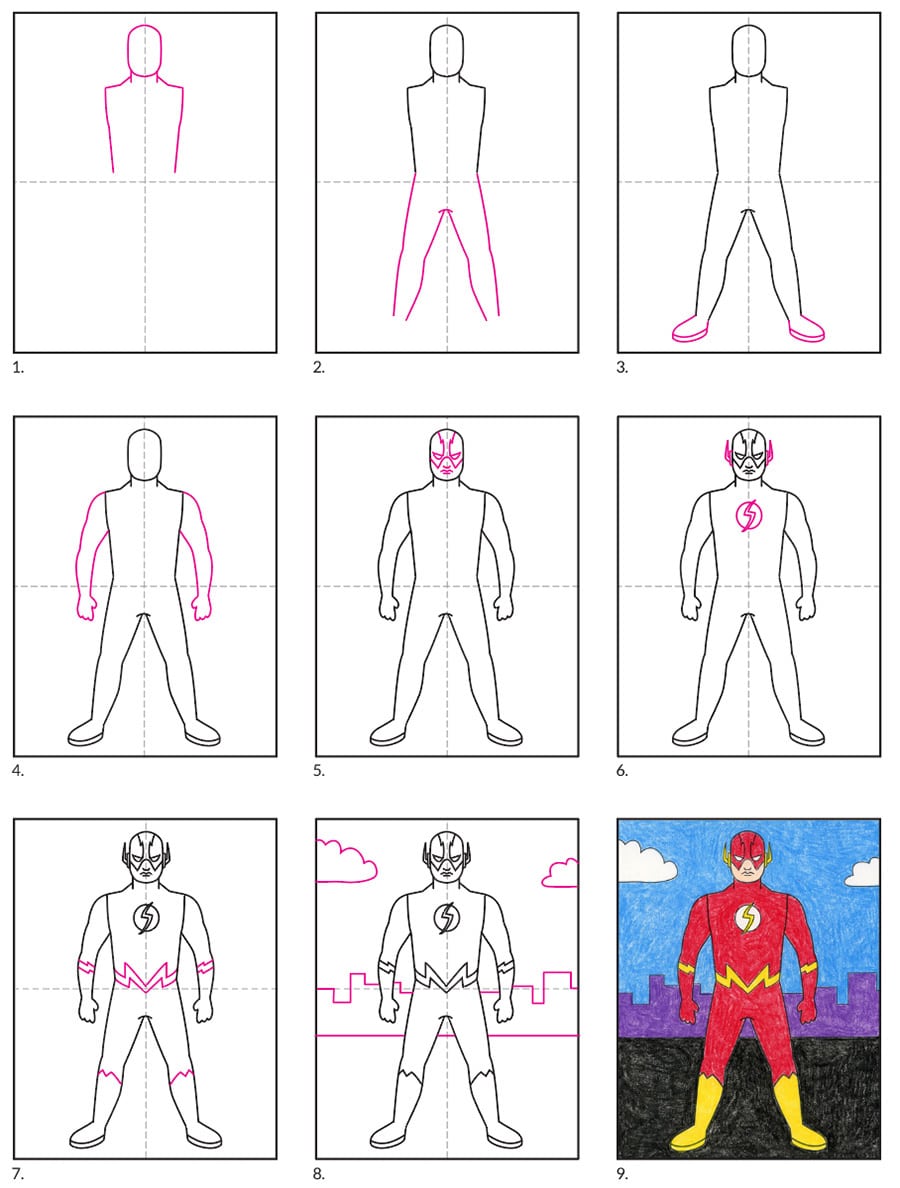
JavaScript: Triggering the Flash
Now, for the JavaScript. I needed a way to start and stop the flashing effect. I wanted a simple function to kick it off. Here’s what I cobbled together:
javascript
function startFlash() {
const flashBar = *(‘flash-bar’);
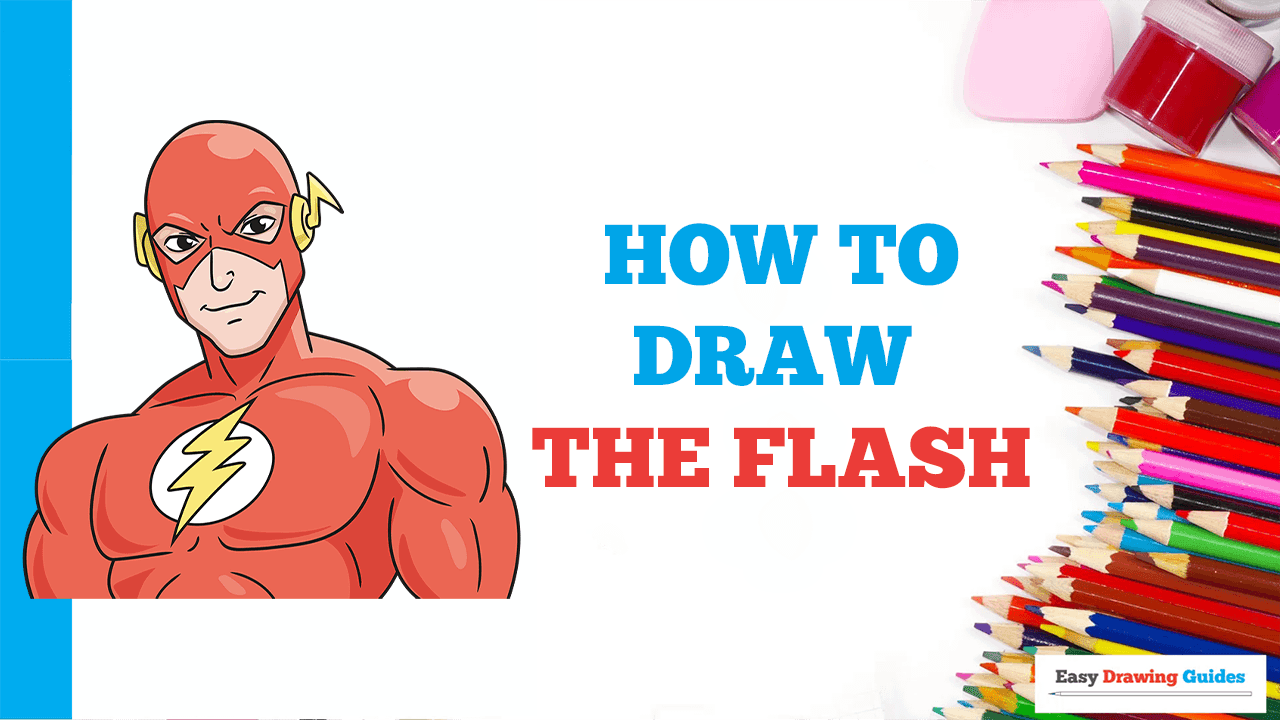
* = ‘0%’; // Reset to start
* = ‘running’;
function stopFlash() {
const flashBar = *(‘flash-bar’);
* = ‘paused’;
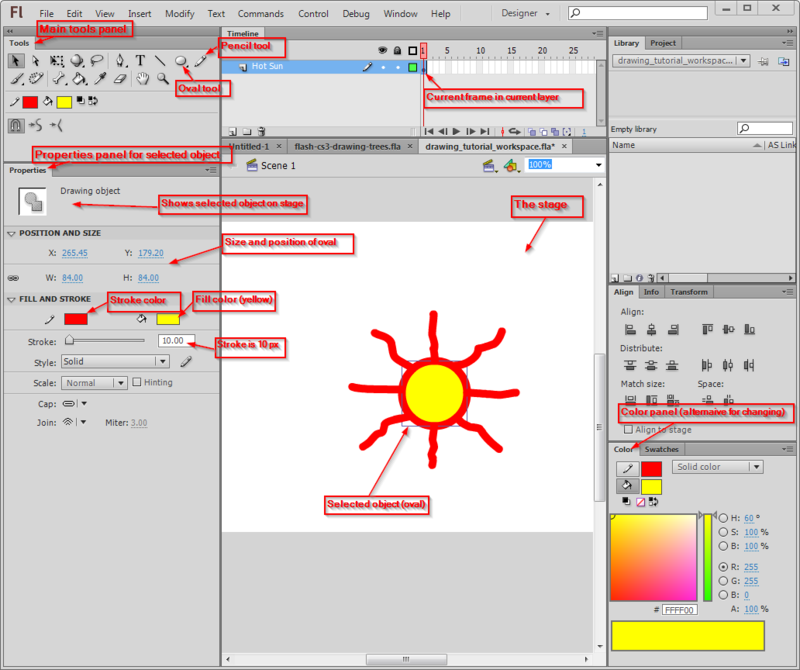
* = ‘0%’; // Optional: Reset to empty
The `startFlash` function resets the `width` to 0% (just to make sure it starts fresh each time) and sets the `animationPlayState` to `running`. The `stopFlash` function pauses the animation. I also added the optional width reset to prevent a weird glitch on some browsers.
Putting It All Together: A Basic Test
To test it out, I added a couple of buttons:
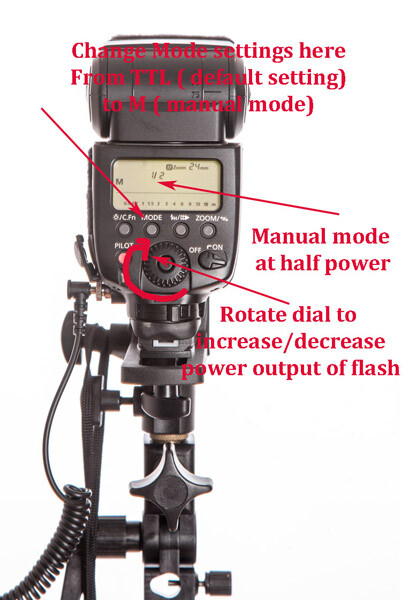
I opened the HTML file in my browser, clicked the buttons, and BAM! It flashed! It wasn’t pretty, but it worked.
Refining It: Making it Smoother
The initial flash was a bit jarring. So, I tweaked the CSS. I adjusted the `animation` property to include an `ease-in-out` timing function:
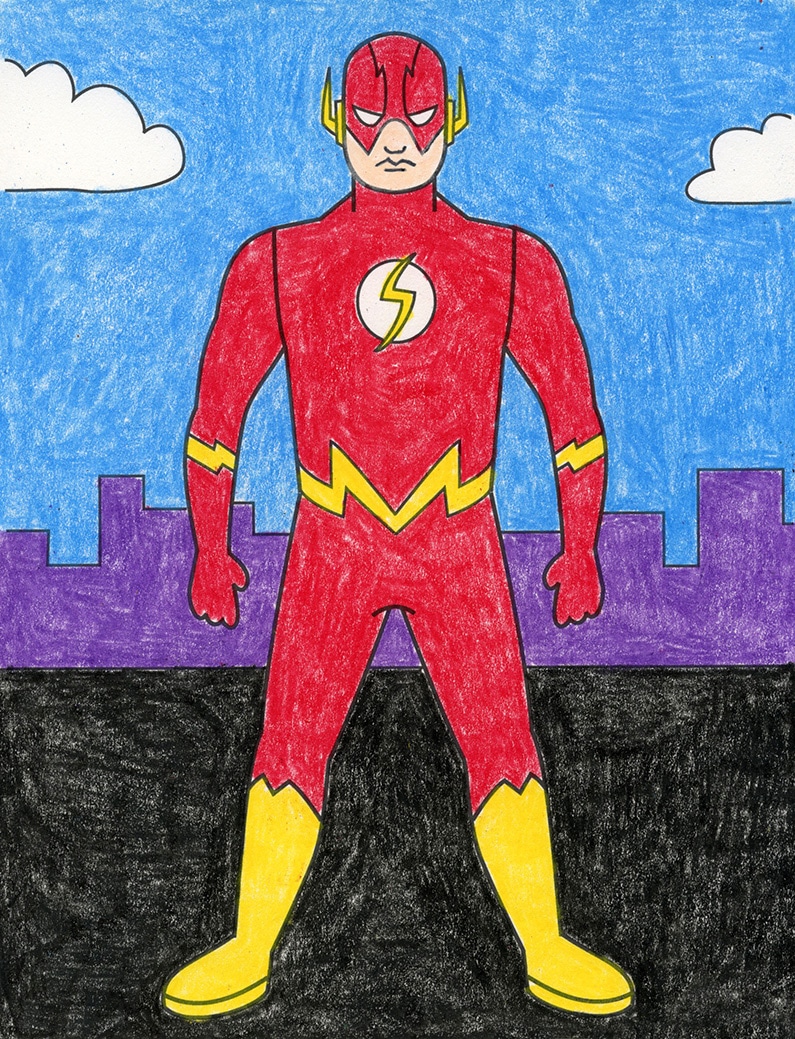
css
animation: flash 1.5s ease-in-out infinite;
This made the animation smoother and less abrupt.
Integrating with Backend Stuff: Real-World Use
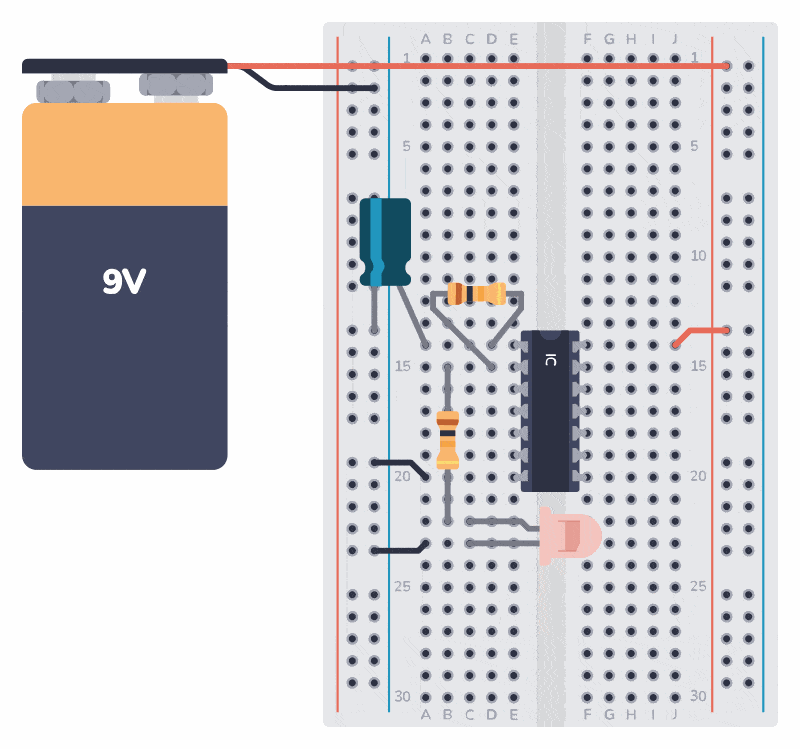
Okay, so the flashy thing was working. Now, the real test: integrating it with actual backend processes. Let’s say I have a function that does some heavy lifting:
javascript
async function doSomethingImportant() {
startFlash(); // Start the flash
// Simulate some work
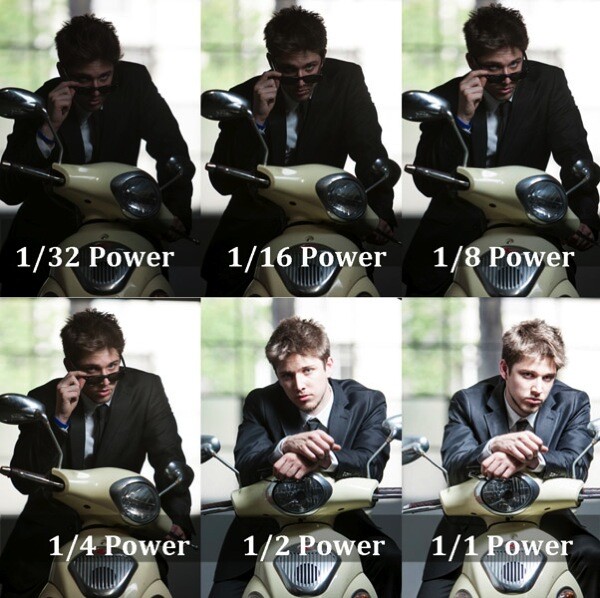
await new Promise(resolve => setTimeout(resolve, 3000));
stopFlash(); // Stop the flash
*(“Done!”);
The key is wrapping the actual work with `startFlash()` and `stopFlash()`. This gives the user visual feedback that something is happening in the background. I found async/await helped prevent the whole interface locking up.
Final Thoughts
This “works flash” isn’t fancy, but it’s simple and effective. It’s a quick way to provide visual feedback to the user when something’s happening in the background. I’m sure there are better ways to do it, but this works for my needs right now. Plus, it was a fun little project to build. Maybe next time, I’ll try to make it a little more configurable, with customizable colors and animation speeds. But for now, I’m happy with it.
- Learned a lot about CSS animations.
- Practiced using JavaScript to manipulate the DOM.
- Found a simple way to provide visual feedback to users.